Modules in Python
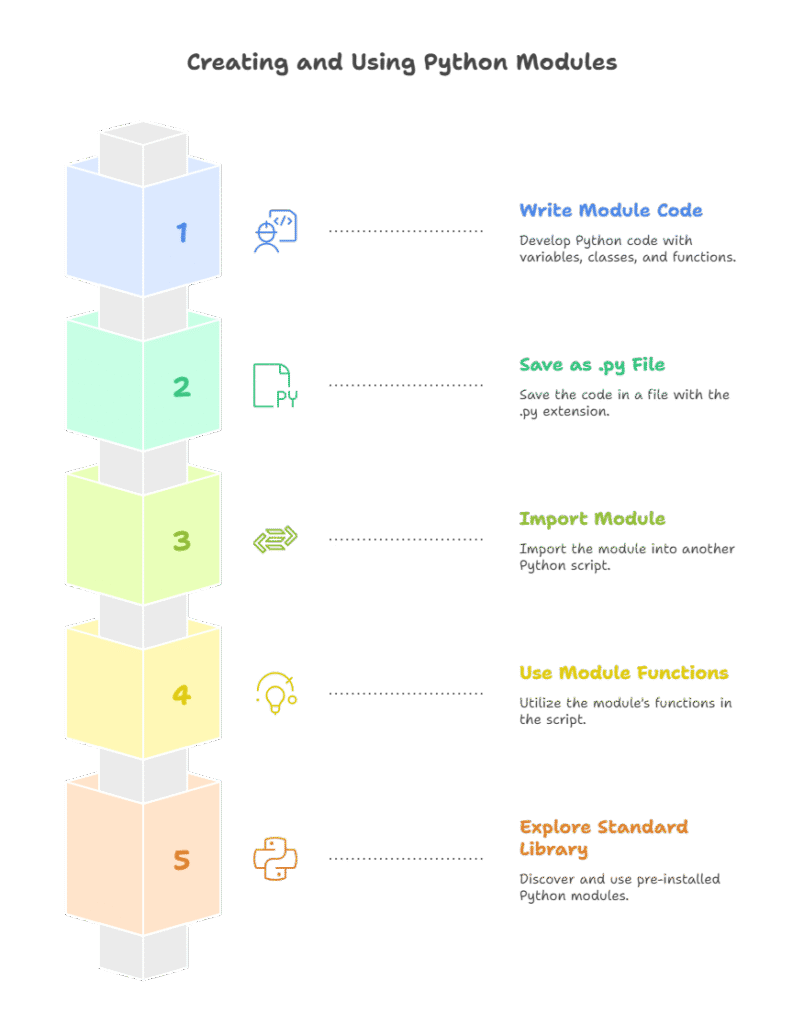
A module in Python is basically a file that contains Python code. This code may contain variables, classes, functions, and executable statements. A module is any program file you generate that has the.py extension. The module is named after the file name without the.py extension.
Code can be logically arranged into self-contained units using modules. They assist in decomposing more complex programs into more manageable, smaller components. There are various advantages to this organisation:
Simplicity: Modules make it simple to concentrate on a specific issue or related group of chores.
Maintainability: It’s simpler to work with and keep smaller files.
Reusability: By allowing code described in a module to be utilized across programs, duplicate code is not necessary.
Scoring: By giving modules their own namespaces, you can avoid naming conflicts between programs or between your code and code that is imported. A module file’s top-level names all become attributes of the imported module object.
Utilising modules is similar to consulting code in a different “chapter” of your software.
How to Use Modules (Importing)
A module’s code must be imported into your running script or application in order to be used. A Python file’s import statements are usually found at the very beginning.
The import statement is most frequently used to import a module:
import module name
Once imported, use the dot notation module name item name to access the items (functions, classes, variables, etc.) specified within that module.
The sqrt (square root) function from the standard math module, for instance, can be used as follows:
Example:
import math
print(math sqrt(25))
Output:
5.0
Pi and other constants are also included in the math module.
Example:
import math
print(math pi)
print(math ceil(5 4))
Output:
3.14159
6
The from keyword can also be used to import particular elements from a module.
from module name import item name
You can refer to the imported object directly using this syntax, omitting the module name prefix.
Example:
from math import sqrt, pi
print(sqrt(16))
print(pi)
Output:
4.0
3.14159
Importing a module or item with an alias using the as keyword is an additional helpful method.
import module name as alias name
# Or
from module name import item name as alias name
This can help to resolve any naming issues or abbreviate lengthy module names.
Example:
import math as m
print(m sqrt(9))
Output:
3.0
Python only imports a module once, even if your code contains several import lines for the same module. It’s customary to import modules from the standard library first, then a blank line, and then import modules that you authored.
Creating New Modules
The process of making your own module is easy. Writing Python code that includes variables, classes, functions, and other elements is all that is required, and it should be saved in a file with the.py extension. When you import the module, the name you specify for the file will be used as its name.
Let’s build a basic unit conversion module, A file called conversions.py should be created.
Example:
# conversions.py
Module with conversion functions.
def cel2fah(c):
Converts Celsius to Fahrenheit.
return (9/5 * c)
def km2mi(km):
Converts kilometers to miles.
return km / 1.60934
The conversions module can now be imported and used in another Python code (such as main app.py) located in the same directory:
Example:
# main app.py
import conversions
celsius temp = 25
# Use the function from the module via dot notation
fahrenheit temp = conversions.cel2fah(celsius temp)
print(f"{celsius temp}°C is {fahrenheit temp}°F")
kilometers = 100
miles = conversions.km2mi(kilometers)
print(f"{kilometers} km is {miles} miles")
Exploring Standard Library Modules
The Python Standard Library comprises a vast array of pre-installed modules for Python. Since this library operates on the “batteries included” tenet, it offers a wealth of practical tools and features without requiring further installations. It is advised to review the standard library documentation, which is accessible online, prior to writing your own code because a solution may already be in place.
Using the built-in dir() and help() functions in an interactive Python session, you can examine the contents of modules:
- The names of the functions, classes, and variables specified in the module are listed by dir(module name).Using help(module name).
- module’s documentation is displayed. The code’s docstrings are used to create documentation.
- module name item name help: displays the module’s documentation for a certain component.
Using some popular standard library modules, let’s examine some examples:
Math Module: Mathematical constants and functions are provided by this module.
Datetime Module: The datetime module offers classes for handling times and dates. It has a class called datetime, as you can see.
operating system Module: Offers features for working with the operating system, including file system capabilities.
Access to system-specific parameters and functions is made possible by the sys module, another essential system module. Sys exit(), for example, can be used to terminate the program.
The scope and scope of the Standard Library are extensive. Studying its documentation is a useful ability for any Python developer.